Perpetual Freefall (Orbit)
The Main Idea
Perpetual free fall, or orbit, is when the force of gravity is equal to the centripetal force of the object’s motion in space. The centripetal force fluctuates based on changes in the object’s tangential velocity. The force of gravity pulls inward on the object, and the centripetal force pushes the object out. When these perpendicular forces are equal, the object will follow a circular path around the massive body which is the source of gravity. The object is in perpetual free fall because gravity is the only force acting upon it, but the velocity continuously prevents the object from just falling towards the earth. This perpetual free fall causes a feeling of weightlessness for the object. In order to enter the state of orbit, the particular conditions described above must be met. The pictures on the right demonstrate this concept. If the tangential velocity is too high, then the object will reach an escape velocity and will continue to travel outward away from the massive object. If the tangential velocity is too low, then the object will fall towards the massive object. For organizations such as NASA or Boeing that utilize satellites, gravity must be overcome to exit the lower atmospheres and make sure their technologies maintain orbit and do not fall back to Earth. This is achieved via perpendicular propulsion and altitude adjustment in lower atmospheres where air resistance is present, or happens when there is a high enough stable velocity outside the Earth's atmosphere.
There are two types of elliptical orbit: closed and open. An open orbit means the orbiting body is moving with at least the escape velocity of the system. Escape velocity is the minimum speed required by the object such that the tangential velocity overcomes the force of gravity allowing the body to be unbound by an orbit. The most common example of this idea is a comet from outside the solar system slinging around a planet and then leaving. A closed orbit occurs when the particle is not moving with at least the escape velocity. This is the traditional case of orbiting. Closed orbits move in the shape of an ellipse except in the very special case of a circular orbit.
A Mathematical Model
Calculating an objects motion is based around the Newton’s law of universal gravitation and the momentum principle which is described by the equation [math]\displaystyle{ {\frac{d\mathbf{p}}{dt}}_{object} = \mathbf{F}_{net} }[/math], where [math]\displaystyle{ \mathbf{F}_{net} }[/math] is equal to the change in the momentum of the object. Gravity is the only force acting on an object which has begun perpetual freefall outside the atmosphere. The gravitational force equation for an object near the surface of the Earth is [math]\displaystyle{ \mathbf{F}_{gravity} = mg }[/math]. Perpetual freefall, however, follows Newton’s Law of Universal Gravitation which is dependent on the mass of both objects, and their distance apart:
- [math]\displaystyle{ \mathbf{F}_{gravity} = \frac{G m_1 m_2}{r^2} \mathbf{\hat r} }[/math], where
- [math]\displaystyle{ G = 6.7 \times 10^{-11} }[/math] (Universal Gravitational Constant)
- [math]\displaystyle{ r = }[/math] The displacement between the two objects
- [math]\displaystyle{ m_1 = }[/math] Mass of object one
- [math]\displaystyle{ m_2 = }[/math] Mass of object two
- [math]\displaystyle{ \mathbf{\hat r} = }[/math] The unit vector in the direction of the displacement between the two masses
Since gravity is the only force acting on the object once it is free from Earth's atmosphere, [math]\displaystyle{ \mathbf{F_{gravity}} }[/math] is equal to the objects net force ([math]\displaystyle{ \mathbf{F_{net}} }[/math]). Once net force is calculated, the momentum principle equation is manipulated so that the object's momentum can be updated as a function of the net force acting on the object:
- [math]\displaystyle{ {\mathbf{p}_{object_{final}} } = \mathbf{p}_{object_{initial}} + \mathbf{F}_{net} {\Delta}{t} }[/math], where
- [math]\displaystyle{ \mathbf{p}_{object} = }[/math] The object's momentum
- [math]\displaystyle{ \Delta t = }[/math] The change in time between measurements/data points
Once the change in momentum is calculated, the velocity of the object can be found using the assumption that:
- [math]\displaystyle{ \mathbf{v}_{average} = \frac{\mathbf{p}_{object_{final}}}{m_{object}} }[/math], where
- [math]\displaystyle{ \mathbf{v} = }[/math] The velocity of the object
- [math]\displaystyle{ m = }[/math] The mass of the object
This velocity value is then used to update the object's position using:
- [math]\displaystyle{ {\mathbf{r}_{object_{final}} } = \mathbf{r}_{object_{initial}} + \mathbf{v}_{average}{\Delta}{t} }[/math], where
- [math]\displaystyle{ \mathbf{r} = }[/math] The object's position
A Computational Model
Below is code written in VPython . The code consists of a setup of the scene, initial conditions and constants, calculation of net force, and an update of momentum, velocity and position. All of these components are expressed visually as a simulation.
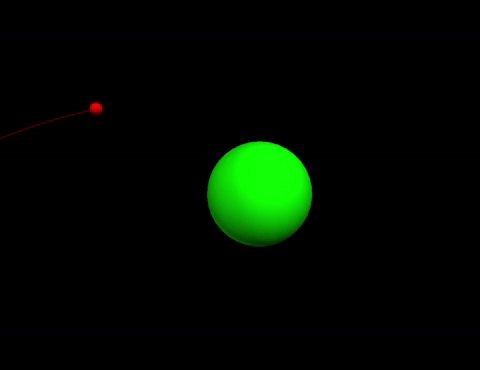
from __future__ import division
from visual import *
from visual.graph import *
SCENE:
scene.width = 1224
scene.height = 960
scene.autoscale = 0
OBJECTS:
Earth = sphere(pos = vector(0,0,0), radius = 4 * 10**6, color = color.green)
scene.range = Earth.radius * 10
ball = sphere(pos = vector(- Earth.radius * 5, Earth.radius + Earth.radius / 25, 0), radius = 5 * 10**5, color = color.red)
trail = curve(color = ball.color)
INITIAL CONDITIONS:
ball.v = vector(5000, 2000, 0)
vhat = ball.v / mag(ball.v)
CONSTANTS:
ball.m = 3000.5
g = 9.8
G = 6.7 * 10**(-11)
mEarth = 6 * 10**(24)
MOMENTUM:
ball.p = ball.m * ball.v
TIME:
t = 05
dt = 1
while t < 10000:
rate(700)
rVecE = ball.pos - Earth.pos
rMagE = mag(rVecE)
FGravMagE = (G * ball.m * mEarth) / (rMagE)**2
rHatE = rVecE / rMagE
FGravE = (FGravMagE) * (-rHatE)
NetForce = FGravE
ball.p = ball.p + NetForce * dt
ball.v = ball.p / ball.m
ball.pos = ball.pos + ball.v * dt
t += dt
trail.append(pos = ball.pos)
if rMagE < Earth.radius:
break
print("time=", t, "s")
print("velocity=", ball.v)
print("position=", ball.pos)
Examples
The simple example requires knowledge of the centripetal force and circular motion.
The middling and difficult examples both require and understanding of energy conservation. It is suggested you come back to these examples after next week's lessons.
Simple
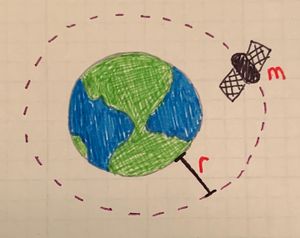
A satellite of mass [math]\displaystyle{ m }[/math] has a circular orbit about the Earth at a radius [math]\displaystyle{ r }[/math] from the surface of the Earth and speed [math]\displaystyle{ v_T }[/math].
- a) Find an expression for the tangential velocity ([math]\displaystyle{ v_T }[/math]) of the satellite?
- From Centripetal Force and Curving Motion, we know that the centripetal force acting on an object in circular motion is:
- [math]\displaystyle{ F_c = \frac{m{v_T}^2}{r} }[/math]
- We also know that the centripetal force is the net perpendicular force acting on an object in an orbiting motion:
- [math]\displaystyle{ F_c = F_{gravity} = mg }[/math]
- Lastly, the radius of the circular orbit is [math]\displaystyle{ r + R_e }[/math], where [math]\displaystyle{ R_e }[/math] is the radius of the Earth.
- Therefore:
- [math]\displaystyle{ mg = \frac{m{v_T}^2}{r+R_e} }[/math]
- Simplifying for [math]\displaystyle{ v_T }[/math] gives:
- [math]\displaystyle{ v_T = \sqrt{g(r+R_e)} }[/math]
Middling
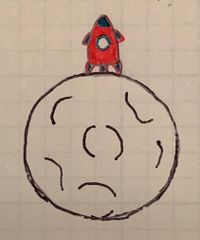
A rocket of mass [math]\displaystyle{ m }[/math] is on the surface of the moon. The mass of the moon is [math]\displaystyle{ M_m = 7.36 \times 10^{22} \ \text{kg} }[/math]. The radius of the moon is [math]\displaystyle{ R_m = 1.74 \times 10^6 \ \text{meters} }[/math].
- a) What is the minimum speed necessary for the rocket to escape the gravitational pull of the moon?
- The minimum speed, also know as the escape velocity ([math]\displaystyle{ v_e }[/math]), can be solved for based on energy considerations.
- The key lies in the fact that at the escape velocity the total energy ([math]\displaystyle{ E }[/math]) of the rocket is just greater than [math]\displaystyle{ 0 }[/math].
- Since we know the kinetic energy of the rocket will be the usual formula, all that is left is to find the potential energy of the rocket.
- Only one force acts on the rocket...gravity. Gravity is governed by:
- [math]\displaystyle{ F_g = - \frac{G m_1 m_2}{r^2} \mathbf{\hat r} }[/math], where
- [math]\displaystyle{ G = 6.7 \times 10^{-11} }[/math]
- [math]\displaystyle{ m = }[/math] The masses
- [math]\displaystyle{ r = }[/math] The displacement between the objects
- [math]\displaystyle{ \mathbf{\hat r} = }[/math] The unit vector in the direction of the displacement
- Taking the classical rule for position dependent forces into account ([math]\displaystyle{ F = - \frac{dU}{dx} }[/math]), we see that:
- [math]\displaystyle{ - F \ dx = dU }[/math], where
- [math]\displaystyle{ U = }[/math] A potential energy function of position for the given force
- Taking the integral of both sides, we see that:
- [math]\displaystyle{ -\int F \ dx = U }[/math]
- We can follow this procedure for the position dependent gravitational force:
- [math]\displaystyle{ - \int F_g \ dr = U_g }[/math]
- [math]\displaystyle{ U_g =- \int_{\infty}^r - \frac{G m_1 m_2}{r^2} \ dr }[/math]
- We choose the bounds so that the 0 of potential energy is at a distance infinitely far away, and that the potential energy is defined as the work done to move the object a distance [math]\displaystyle{ r }[/math] towards the body exerting the gravitational force.
- Evaluating this integral gives:
- [math]\displaystyle{ G m_1 m_2 \int_{\infty}^r \frac{1}{r^2} \ dr = (G m_1 m_2) \times - \left[\frac{1}{r}\right]_{\infty}^r = -Gm_1m_2 \times \left[ \frac{1}{r} - \frac{1}{\infty}\right] = -\frac{Gm_1m_2}{r} }[/math]
- Therefore the potential energy function for an object feeling the gravitational field of the moon is:
- [math]\displaystyle{ U_g = -\frac{GM_m m}{r} }[/math]
- Since there is no heat intake or loss considered in this question, we can state the total energy of the rocket as:
- [math]\displaystyle{ E = K + U_g }[/math]
- And set this equal to [math]\displaystyle{ 0 }[/math]:
- [math]\displaystyle{ E = K + U_g = 0 }[/math]
- Plugging in known expressions for [math]\displaystyle{ K \ \text{and} \ U_g }[/math] gives:
- [math]\displaystyle{ \frac{1}{2} m{v_e}^2 - \frac{GM_m m}{R_m} = 0 }[/math]
- We begin simplifying for the escape velocity by moving the potential energy term to the other side:
- [math]\displaystyle{ \frac{1}{2} m {v_e}^2 = \frac{GM_m m}{R_m} }[/math]
- Next, with some quick algebra, we find the minimum velocity needed to escape the moon is:
- [math]\displaystyle{ v_e = \sqrt{\frac{2 G M_m}{R_m}} }[/math]
- Plugging in numbers gives:
- [math]\displaystyle{ v_e = \sqrt{\frac{2 \times 6.7 \times 10^{-11} \times 7.36 \times 10^{22}}{1.74 \times 10^6}} = 2380.8 \ \frac{m}{s} }[/math]
In compliance with MIT OpenCourseWare privacy and terms, the previous example maybe found here [1]
Difficult
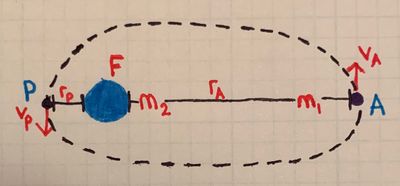
A satellite of mass [math]\displaystyle{ m_1 }[/math] is in an elliptical orbit around a planet of mass [math]\displaystyle{ m_2 }[/math], whose center is at F, one of the two foci of the ellipse ([math]\displaystyle{ m_2 \gt \gt m_1 }[/math]). P is the closest approach of the two masses and is a distance [math]\displaystyle{ r_p }[/math] from the foci F. The satellite's speed at the point P is [math]\displaystyle{ v_p }[/math]. The two ,asses are furthest apart at point A, which is a distance [math]\displaystyle{ r_A = 5r_p }[/math].
- a) What is the speed of the satellite at point A ([math]\displaystyle{ v_A }[/math])?
- The key to this question is angular momentum ([math]\displaystyle{ L }[/math]). For any orbit with no external forces, angular momentum will be conserved. Therefore we know the angular momentum of the satellite at point P should equal the angular momentum of the satellite at point A:
- [math]\displaystyle{ L_p = L_A }[/math]
- It is known that in general the angular momentum of an object is equal to its radius of orbits times its mass times its tangential speed:
- [math]\displaystyle{ L = rmv }[/math]
- Therefore:
- [math]\displaystyle{ r_p m_1 v_p = r_A m_1 v_A }[/math]
- Substituting [math]\displaystyle{ 5r_p \ \text{for} \ r_A }[/math] gives:
- [math]\displaystyle{ r_p m_1 v_p = 5r_p m_1 v_A }[/math]
- Cancelling terms leads to:
- [math]\displaystyle{ v_A = \frac{r_p}{5} }[/math]
- b) What is the energy of the satellite ([math]\displaystyle{ E_{satellite} }[/math])?
- Since we aren't taking heat into consideration, the energy we wish to find is the kinetic energy plus the potential energy:
- [math]\displaystyle{ E = K + U }[/math]
- The only force working on the satellite is gravity, so the only potential energy is due to gravity, which we found a potential energy function for in the middling example:
- [math]\displaystyle{ E = K - \frac{Gm_1m_2}{r} = \frac{1}{2}mv^2 - \frac{Gm_1m_2}{r} }[/math]
- We know energy should be conserved in the elliptical orbit, so the energy at point P should equal the energy at point A:
- [math]\displaystyle{ E_p = E_A }[/math]
- Which is:
- [math]\displaystyle{ \frac{1}{2}m_1 {v_p}^2 - \frac{Gm_1m_2}{r_p} = \frac{1}{2}m_1 {v_A}^2 - \frac{Gm_1m_2}{r_A} }[/math]
- Plugging in [math]\displaystyle{ 5v_A \ \text{for} \ v_p \ \text{and} \ 5r_p \ \text{for} \ r_A }[/math] gives:
- [math]\displaystyle{ \frac{1}{2}m_1 {(5v_A)}^2 - \frac{Gm_1m_2}{r_p} = \frac{1}{2}m_1 {v_A}^2 - \frac{Gm_1m_2}{5r_p} }[/math]
- Moving terms around creates:
- [math]\displaystyle{ \frac{1}{2}m_1 {(5v_A)}^2 - \frac{1}{2}m_1 {v_A}^2 = \frac{Gm_1m_2}{r_p} - \frac{Gm_1m_2}{5r_p} }[/math]
- And combining like terms and factoring gives:
- [math]\displaystyle{ 12m_1 v_A^2 = \frac{4Gm_1m_2}{5r_p} }[/math]
- Cancelling [math]\displaystyle{ m_1 }[/math] on each side dividing each side by 4 gives:
- [math]\displaystyle{ 3{v_A}^2 = \frac{Gm_2}{5r_p} }[/math]
- Thus:
- [math]\displaystyle{ v_A = \sqrt{\frac{Gm_2}{15r_p}} }[/math]
- We can plug this value of [math]\displaystyle{ v_A }[/math] back into the energy equation for point P, since [math]\displaystyle{ 5v_A = v_p }[/math] to get:
- [math]\displaystyle{ E_p = \frac{1}{2}m_1 \left(5\sqrt{\frac{Gm_2}{15r_p}}\right)^2 - \frac{Gm_1m_2}{r_p} }[/math]
- Which equals:
- [math]\displaystyle{ E_p = \frac{25}{2} \frac{Gm_1m_2}{15r_p} - \frac{Gm_1m_2}{r_p} }[/math]
- [math]\displaystyle{ E_p = \frac{Gm_1m_2}{r_p} \left(\frac{5}{6} - \frac{6}{6}\right) }[/math]
- Therefore the energy of the satellite is:
- [math]\displaystyle{ E = -\frac{1}{6}\frac{Gm_1m_2}{r_p} \lt 0 }[/math]
- Note the energy of the satellite is less than 0 as it should be for a satellite in orbit.
In compliance with MIT OpenCourseWare privacy and terms, the previous example maybe found here [2]
Connectedness
Perpetual freefall (orbit) from a frame of reference that it's possible for an object to fall past the curvature of the Earth comparative to an object merely orbiting around the Earth as a constant velocity is an interesting concept that gives a more accurate representation of how objects actually orbit the Earth. The industrial applications of this principle are seen everyday, as companies such as [Boeing], [NASA], and [SpaceX] must use the most basic form of these principles and expand upon it to achieve space and outer atmospheric flight.
Clear understanding of different types of orbits is vital for a number of industries. Many applications of orbitals include spy satellites, communication satellites, space tourism, and space colonization. Without an understanding of orbits everyday things such as satellite TV and cellular communication would not be possible. These ideas may be particularly interesting for students studying aerospace engineering, material science engineering, and mechanical engineering. By understanding off-earth interactions, humans are better equipped to control expansion.
History
The planetary orbits had been observed by astronomers for decades before the first theories on planetary motion were concieved. [| Johannes Kepler] was one of the first to observe and prove that the motions that were being observed were elliptical in nature. From this, [Sir Isaac Newton] was able to combine Kepler's principles with his own theories of motion and that Keplar's theories could be proven by his own theory of gravitation. This derivation led to the relation of a planet's mass to the gravitational force acting on an object which became Newton's gravitational equation used in the calculation of orbits.
Einstein later disproved Newton's methodology as the best model for orbital motion as he explained gravity was due to curvature in space time. As a result of this, astronomers recognized Newtonian physics did not provide the best model of this phenomena. Although Einsteins realistic motion explains orbits the best, Newtonian methods still provide enough accuracy to be used for short term purposes due to their relative simplicity to Einstein's ideas.