VPython Object
A VPython Object is a representation of data in a specific in VPython both visually and numerically. Each object represents data through its attributes, specific characteristics assigned to each individual object. Objects play an essential role in the necessary knowledge for the coding portion of PHYS 2211 and 2212, as most of your programs will require the extensive usage and manipulation of objects. This page is intended to provide you a background on these objects, especially if you have no prior knowledge of coding or VPython.
In this class, you should know how to create sphere objects and set their positions, and how to use arrows to show forces, fields, velocities, etc.
The Main Idea
Objects in VPython are intended to represent data in ways that can be easily visualized and understood by both the user and the computer. Each object has a type it belongs to, which determines its default attributes, as well as how the computer will choose to display it when the program runs. Objects must be uniquely named if you wish to refer to them again later in your program, and any graphical object you create (spheres, boxes, curves, arrows, etc.) continue to exist for as long as your program remains running - VPython will continue to display them regardless of where they are positioned. If you change an attribute of an object, such as its position or color, VPython will automatically display the object in its new location and/or with its new color.
For example, let's say you create a sphere called 'ball' - by default, ball has attributes pre-assigned to it, such as ball.pos (the .pos attribute signifies the position of the sphere) or ball.color (the .color attribute allows you to change the color of the sphere). Also, in addition to the preset attributes, you can also create new ones. Besides the position and radius, you can also create attributes for mass (ball.mass), velocity (ball.vel), momentum (ball.momentum), or anything else you see fit. Of course, not all attributes need to be added manually. They can be left blank and filled in with a default value which the program automatically assigns them
Basics
To make an object and visualize it, it is required to import functions by writing following lines on the top of the code. If you are using Glowscript, these imports are not required.
from __future__ import division from visual import *
Then, by writing following line on your code, you can instantiate
[variable name] = [object type]([attribute1].[attribute1 type], ... ,[attribute n].[attribute n type])
For an object of type sphere, attributes might include position (pos), color, and size. You can also add attributes such as charge or material. For an arrow, typical attributes are position (pos), the axis vector, and color.
You can also change attributes of the certain objects after they have been created.
[variable name].[attribute] = [new attribute type]
Attributes
Many objects in VPython carry common attributes regardless of their type. This section will cover the attributes you will be utilizing the most in this class.
Position
The position attribute is common to almost every single object, and is probably the most important of the attributes. It determines the position of the object's center (in the case of spheres, boxes, etc.) or the object's endpoint (arrows, curves, etc.). It is of the vector type. To access and/or edit this attribute, it can be accessed using objectName.pos. Returning to the example from the above section, we can access the position of our sphere called 'ball' by typing
ball.pos
This would return the position of the ball in vector form, or allow you to refer to said position in calculations.
In addition, because this is a vector, the position attribute has three components of its own, which can be accessed by typing:
ball.pos.x ball.pos.y ball.pos.z
depending on the desired component of the position. The same rules apply as to ball.pos, however they are returned as single numbers instead of as a vector.
To set the position of an object, one must first set the type of the object and then type pos([vector coordinates]). For instance, if we wanted to create an object called ball centered at (4,2,0), we would type
ball = objectType(pos(4,2,0))
Color
The color attribute defines the object's color when it appears in the display window. This attribute is not important for data but more for display - it is simply to distinguish objects from one another, which is especially useful if you are working with a multitude of objects at once.
Color can be assigned in one of two ways. The first way it can be assigned is through a preset color. The preset colors in VPython are blue, orange, green, red, purple, brown, pink, gray, olive, and cyan - any of these can be inserted to create the desired color. For instance, the syntax for creating a red color is:
color = color.red
Another way of assigning color is through an RGB vector, an additive form of assigning color. Red, green and blue layers are added together in various saturations and opacities to reproduce a broad array of colors. The vector contains three numbers from 0 to 255 - The first number represents the red, the second represents the green, and the third represents the blue. The higher the value of the number, the more it resembles that particular color. For instance, if we wanted to assign cyan to color, we would type
color = (0, 255, 255)
To set the color of an object, one must first set the type of the object and then set the color within the parentheses. For instance, if we wanted to create an object called ball which was cyan, we would type either
ball = objectType(color = color(cyan)) ball = objectType(color = (0,255,255))
Axis
The axis attribute is important specifically for arrows, as it determines the direction which the arrow points and how long it is. For instance, if you wanted to create an arrow parallel to the x-axis with a length of 5, the syntax could be:
myArrow = arrow(pos=vec(5, 0, 0), axis=vec(1, 1, 1), color=color.green)
meaning the arrow points 5 units along the x axis and is perpendicular to the y and z axes. You will often use arrows to represent forces and fields, where the position will be the observation point, and the axis will the vector value of the force/field/etc.
The axis attribute also determines orientation of other objects, such as rings or cylinders, but arrows are the only object whose size is specifically tied to the axis attribute.
Setting Attributes in a Loop
Sometimes, the position vector will be given, and will be easy to set. In other cases, you may want to create, say 12 spheres in a circle, all a distance 2 from the origin. A quick way to do this is by by using a loop or a list.
For example:
i = 0 while i < 2*pi: sphere(pos=vec(2*cos(i), 2*sin(i), 0), radius=0.5, color=color.green) i = i + 2*pi/12
This would create 12 green spheres of radius .5 in a circle 2 units from the origin:
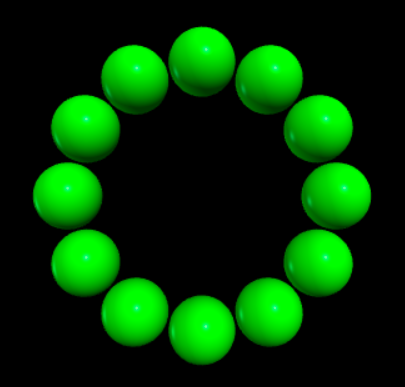
Material/Texture
One of the main purposes of VPython programming is to help people's understanding on a physical principle or phenomenon by displaying an animation describing the principle or phenomenon. To achieve this, various objects are used in the program, and various attributes such as color and shape are used to distinguish them. Material is also one of that attributes, which does not change the essence of the phenomenon but helps people's understanding on the phenomenon.
Basic Materials
Wood
materials.wood
woodsphere = sphere(pos = (-2,0,0), radius = 1, material = materials.wood) woodbox = box(pos = (2,0,0), length=1, height=1, width=1, material = materials.wood)
Rough
materials.rough
roughsphere = sphere(pos = (-2,0,0), radius = 1, material = materials.rough) roughbox = box(pos = (2,0,0), length=1, height=1, width=1, material = materials.rough)
Marble
materials.marble
marblesphere = sphere(pos = (-2,0,0), radius = 1, material = materials.marble) marblebox = box(pos = (2,0,0), length=1, height=1, width=1, material = materials.marble)
Plastic
materials.plastic
plasticsphere = sphere(pos = (-2,0,0), radius = 1, material = materials.plastic) plasticbox = box(pos = (2,0,0), length=1, height=1, width=1, material = materials.plastic)
Earth
materials.earth
earthsphere = sphere(pos = (-2,0,0), radius = 1, material = materials.earth) earthbox = box(pos = (2,0,0), length=1, height=1, width=1, material = materials.earth)
Diffuse
materials.diffuse
diffusesphere = sphere(pos = (-2,0,0), radius = 1, material = materials.diffuse) diffusebox = box(pos = (2,0,0), length=1, height=1, width=1, material = materials.diffuse)
Emissive Material which looks like it glows
materials.emissive
emissivesphere = sphere(pos = (-2,0,0), radius = 1, material = materials.emissive) emissivebox = box(pos = (2,0,0), length=1, height=1, width=1, material = materials.emissive)
Unshaded Material which does not affected by lighting
materials.unshaded
unshadedsphere = sphere(pos = (-2,0,0), radius = 1, material = materials.unshaded) unshadedbox = box(pos = (2,0,0), length=1, height=1, width=1, material = materials.unshaded)
Shiny
materials.shiny
shinysphere = sphere(pos = (-2,0,0), radius = 1, material = materials.shiny) shinybox = box(pos = (2,0,0), length=1, height=1, width=1, material = materials.shiny)
Chrome
materials.chrome
chromesphere = sphere(pos = (-2,0,0), radius = 1, material = materials.chrome) chromebox = box(pos = (2,0,0), length=1, height=1, width=1, material = materials.chrome)
Blazed
materials.blazed
blazedsphere = sphere(pos = (-2,0,0), radius = 1, material = materials.blazed) blazedbox = box(pos = (2,0,0), length=1, height=1, width=1, material = materials.blazed)
Silver
materials.silver
silversphere = sphere(pos = (-2,0,0), radius = 1, material = materials.silver) silverbox = box(pos = (2,0,0), length=1, height=1, width=1, material = materials.silver)
BlueMarble Earth with clouds
materials.BlueMarble
BlueMarblesphere = sphere(pos = (-2,0,0), radius = 1, material = materials.BlueMarble) BlueMarblebox = box(pos = (2,0,0), length=1, height=1, width=1, material = materials.BlueMarble)
Bricks
materials.bricks
brickssphere = sphere(pos = (-2,0,0), radius = 1, material = materials.bricks) bricksbox = box(pos = (2,0,0), length=1, height=1, width=1, material = materials.bricks)
Creating your own texture
Making a text from a photo
You can create a texture with a image file, using PIL, the Python Image Library. To do this,PIL must be installed on the computer and the function "Image" should be imported.
from visual import * import Image # Must install PIL
And the basic syntax is
name = "buzz" width = 64 # must be power of 2 height = 64 # must be power of 2 im = Image.open(name+".jpg") #print(im.size) # optionally, see size of image # Optional cropping: #im = im.crop((x1,y1,x2,y2)) # (0,0) is upper left im = im.resize((width,height), Image.ANTIALIAS) materials.saveTGA(name,im)
You can use the texture "im", which is created with an image file "buzz", using following code
tex = materials.texture(data=im, mapping="rectangular") buzzbox = box(pos = (2,0,0), length=1, height=1, width=1, material = tex) buzzsphere = sphere(pos = (-2,0,0), radius = 1, material = tex)
Other Attributes
There are other attributes which are also important but not as essential (or common) as position/color/axis, such as radius and make_trail, among others. Most of these attributes are self-explanatory or will not be used in the course, so if you would like full descriptions of these attributes, refer to the VPython Documentation.
Common Objects
In order to display and understand your code effectively, there are a certain few objects which will be essential, especially for this class.
Sphere
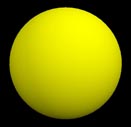
Spheres are the most basic but also very useful objects. A sphere object is a representation of a sphere with a set radius, position, and color. Spheres are commonly used to represent particles in assignments and labs, and are generally assigned a velocity / momentum / etc. in problems. Spheres generally have three properties which should be set when creating them - radius, position, and color, in any order within the parentheses. Only the position is required - note that the position attribute for a cylinder, arrow, cone, and pyramid corresponds to one end of the object, whereas for a sphere it corresponds to the center of the object. If a radius and color are not specified, VPython will set the radius to 1 and the color to the current foreground color.
For instance, if we wanted to create a yellow sphere at the origin with a radius of ten, we would type
yellowSphere = sphere(radius = 10, pos = vector(0,0,0), color=color.yellow)
Arrow
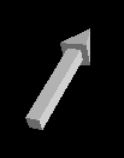
Arrows are just as multipurpose as spheres, if not more so. In this class, arrows will be used to represent vector quantities visually. For instance, you can use arrows to show the velocity/acceleration/momentum of a particle, the electric field direction around a charged particle, etc. Arrow objects are created with a position which represents the starting point of the arrow - not the center - and an axis which represents the arrow's direction and length. Both the position and the length are vector quantities. The color is again not essential as VPython will set it to a default value if left out. The arrow object itself features a straight box-shaped shaft with a 3d pyramid-shaped arrowhead at one end.
For instance, to create an white arrow based at (4,2,0) pointing in the direction of (-2,2,-4), we would type
nameArrow = arrow(pos=(4,2,0), axis=(-2,2,-4), color=color.white)
Box
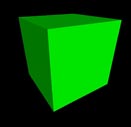
Boxes will feature more in Physics 1 during the kinematics and dynamics sections. While the position attribute is the center of the box as with a sphere, the other attributes of boxes are more complex than spheres or arrows, and particular heed must be paid to the axis attribute, as the box's rotation and orientation depends on it. The axis attribute sets the direction of the length of the box, assuming the length, width and height of the box are set before. If they are not given, the length is automatically set to the magnitude of the axis vector.
To create a green cube with its corner at the origin and a side length of 5 (pictured), we would type
greenbox = box(pos=(2.5, 2.5, 2.5), length=5, height=5, width=5)
The axis attribute can also be added to tilt the box by changing the angle of the length. For example, this would cause the box from the previous step to be tipped at a 45 degree angle:
tippedbox = box(pos=(2.5, 2.5, 2.5), axis(1,1,0), length=5, height=5, width=5)
The box can also be rotated around its own axis by changing which way is "up" for the box, by specifying an up attribute for the box that is different from the up vector of the coordinate system. For instance, this would take the tipped box from the previous step and rotate it so that the top face of the box is perpendicular to the vector in question.
rotatedbox = box(pos=(2.5, 2.5, 2.5), axis(1,1,0), length=5, height=5, width=5, up=(2, 3, 1))
Cylinder
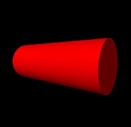
Cylinders are useful wherever a rod comes into play. For instance, in Physics 2, you may see problems regarding charged rods or something of the sort. The cylinder's attributes are a bit different and incorporate elements of most of the other shapes. As such, knowing cylinder syntax gives you a good basis for any object you need to create. The position vector determines the center of the cylinder's base (similar to a cone's pos attribute) and not the center of the object, while the axis determines the direction and length of the cylinder (similar to the arrow's axis attribute). The radius is self-explanatory.
For example, to make a red cylinder with the base centered at (4,1,3) which is 6 units tall, has a radius of 2, and is laying parallel to the x-axis, you would type
cyl = cylinder(pos=(4,1,3), axis=(6,0,0), radius=2, color=color.red)
Other
Aside from the four main objects listed above, there are many more objects which can be used in your programs. You will not need the majority of them for this class, but they do exist, and you may want to learn about them and their attributes. Visit the VPython Documentation for more information on the following objects:
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
Examples
Simple
Create a green sphere named "greenSphere" with a radius of 5 located at the origin.
Solution |
greenSphere = sphere(radius=5, pos=vector(0,0,0), color=color.green)
|
Middling
Create two objects.
- Object 1: A magenta sphere of radius 3 named sphereOne at the position (9,0,8)
- Object 2: A cyan arrow named arrowTwo that points from the origin to the center of sphereOne.
Solution |
sphereOne = sphere(radius=3.5, pos=vector(9,0,8), color=color.magenta)
|
---|
position = vector(0,0,0)
|
arrow(pos=position, axis=(sphereOne.pos - position), color = color.cyan)
|
Note: the position vector is used in the second line, because arrowTwo.pos cannot be called yet. The arrow has yet to be created - it is created in line 3. |
Difficult
Create three objects.
- Object 1: Sphere, radius of 2, located at (-4,-3,8)
- Object 2: Sphere, radius of 1, located at (6,11,0)
- Object 3: Curve, starting at origin, moving to first sphere, then to second
Solution |
sphereOne = sphere(radius = 2, pos=vector(-4,-3,8))
|
---|
sphereTwo = sphere(radius = 1, pos=vector(6,11,0))
|
curveOne = curve(color=color.red, pos = (0,0,0))
|
curveOne.append(sphereOne.pos)
|
curveOne.append(sphereOne.pos)
|
Applying Texture
Trinket Link [2]
VPython code
from __future__ import division from visual import * from visual.graph import * scene.width=1024 scene.height=760
# CONSTANTS G = 6.7e-11 mEarth = 6e24 mcraft = 15000 deltat = 60 t = 0 mMoon = 7e22 scale = 800000 c=2.99792e8
#OBJECTS AND INITIAL VALUES Earth = sphere(pos=vector(0,0,0), radius=15e6, material = materials.BlueMarble) # Add a radius for the spacecraft. It should be BIG, so it can be seen. craft = sphere(pos=vector(-60160000,-192000,0), radius=2e6, material = materials.marble) Moon = sphere(pos=vector(4e8,0,0), radius=1.75e6, material = materials.marble) vcraft = vector(735,2849,0) pcraft = mcraft*vcraft
trail = curve(color=craft.color) # This creates a trail for the spacecraft #scene.autoscale = 1000000 # And this prevents zooming in or out
pscale = Earth.radius / mag(pcraft) fscale = Earth.radius/ ((G*mEarth*mcraft)/mag(craft.pos-Earth.pos)**2) dpscale = 500 * Earth.radius/mag(pcraft)
r_EarthMoon = Moon.pos - Earth.pos #Relative position vector from Earth to Moon r_EarthMoon_mag = mag(r_EarthMoon) F_EarthMoon_mag = (G*mcraft*mEarth)/(r_EarthMoon_mag**2) r_EarthMoon_hat = r_EarthMoon/r_EarthMoon_mag
Force_EarthMoon = (-r_EarthMoon_hat)*F_EarthMoon_mag #Force on Moon due to Earth pMoon = Force_EarthMoon / deltat
# CALCULATIONS print("p=", pcraft) while t < (10e10): rate(500) # This slows down the animation (runs faster with bigger number) rE = craft.pos - Earth.pos rEmag = mag(rE) FEmag = (G*mcraft*mEarth)/(rEmag**2) rEhat = rE/rEmag FEnet = (-rEhat)*FEmag
rM = craft.pos - Moon.pos rMmag = mag(rM) FMmag = (G*mcraft*mMoon)/(rMmag**2) rMhat = rM/rMmag FMnet = (-rMhat)*FMmag Fnet = FMnet+FEnet
pcraft_i = pcraft + vector(0,0,0) pcraft_ii = mag(pcraft_i) pcraft = pcraft + ((Fnet)*deltat) pcraft_f = pcraft pcraft_ff = mag(pcraft) vavg = pcraft/mcraft speedcraft = mag(vavg) craft.pos = craft.pos + vavg*deltat
Fnet_tangent = ((pcraft_ff-pcraft_ii)/deltat)*(pcraft/mag(pcraft))*pscale Fnet_perp = Fnet - Fnet_tangent
r_EarthMoon = Moon.pos - Earth.pos #Relative position vector from Earth to Moon r_EarthMoon_mag = mag(r_EarthMoon) F_EarthMoon_mag = (G*mcraft*mEarth)/(r_EarthMoon_mag**2) r_EarthMoon_hat = r_EarthMoon/r_EarthMoon_mag r_craftMoon = Moon.pos - craft.pos#Relative position vector from spacecraft to Moon r_craftMoon_mag = mag(r_craftMoon) F_craftMoon_mag = (G*mcraft*mEarth)/(r_craftMoon_mag**2) r_craftMoon_hat = r_craftMoon/r_craftMoon_mag
Force_EarthMoon = (-r_EarthMoon_hat)*F_EarthMoon_mag #Force on Moon due to Earth Force_craftMoon = (-r_craftMoon_hat)*F_craftMoon_mag#Force on Moon due to spacecraft Fnet_Moon = Force_EarthMoon + Force_craftMoon #Net force on Moon
momentum_Moon = pMoon + Fnet_Moon*deltat#Update momentum of Moon vavg_Moon = momentum_Moon/mMoon Moon.pos = Moon.pos + vavg_Moon * deltat #Update momentum of Moon
trail.append(pos=craft.pos) t = t+deltat pcraft = pcraft_f deltap = pcraft - pcraft_i
Connectedness
How is this topic connected to something that you are interested in?
- I quite enjoy coding myself on my own time; I have taken a couple online classes outside of Tech on Java and a class here on MATLAB. However, Python and its variants are still far and away my favorite languages to code in, and the one I am most knowledgeable in. I believe the inclusion of VPython in the Physics curriculum is overall a benefit to us students as it allows us to both learn the physics and visualize them by creating the models we do in Labs on VPython.
How is it connected to your major?
- As a Business Admin major I'll be doing IT Management, which requires me to have an understanding of computer systems at the very least. While VPython may not be quite what I need in the future, it is still a good basis in learning the ins and outs of a major programming language long before I have to delve into serious CS training - plus it lets me keep my coding skills sharp!
Is there an interesting industrial application?
- Of course! Whereas the simplicity of Python/VPython have made it effective to illustrate simple physics as we do, it's also powerful elsewhere. VPython is being used by some as a simulation tool for robotics among other things, while Python itself is the backbone for thousands of industrial softwares and applications. In addition, agencies such as NASA use Python and VPython in their labs - I used both programs during my two separate internships at NASA Glenn Research Center in Cleveland, OH.
See Also
VPython - VPython's biography, if you will
VPython Basics - Especially if you're totally new to coding as a whole, this page will bolster your learning
VPython Functions - Working with functions, assuming you understand the basics of coding
VPython Loops - The next step in VPython code knowledge after functions - using loops
VPython Troubleshooting - Having problems? Check here!
References
http://vpython.org/contents/docs/index.html
http://vpython.org/contents/docs/color.html
http://vpython.org/contents/docs/primitives.html
http://vpython.org/contents/docs/materials.html
http://guigui.developpez.com/cours/python/vpython/en/?page=object