Inclined Plane
Claimed by Catherine Grey Spring 2017
The Main Idea
We typically know an inclined plane as a flat surface that is higher on one end aka a big triangle. Inclined planes are commonly used to move objects to a higher or lower place. These slopes help to lessen the force needed to move an object but do require the object to move a greater distance, the hypotenuse of the triangular plane. Some examples of inclined planes include ramps, stairs, wedges (such as a door stopper), or even mountains which people sled down. To solve an equation with an inclined plane forces may be taken into account or the energy principle if the object is moving or has a rotation that is on the inclined plane.
A Mathematical Model
This figure illustrates most of the forces that could act on an inclined plane. Depending on the question not all will be present, specifically friction.
Variables:
θ = Angle of the plane to the horizontal
g = Acceleration due to gravity
m = Mass of object
N = Normal force (perpendicular to the plane)
Ff = frictional force of the inclined plane (sometimes it is omitted on test problems)
mgSinθ = A force parallel to the plane (mgSinθ > Ff the body slides down the plane)
mgCosθ = A force acting into the plane (opposite to N)
Fnetparallel = The net parallel force acting on the object
Fnetperpendicular = The net perpendicular force acting on the object
Equations:
- [math]\displaystyle{ \ F_{net} = F_{net//} + F_{netperp} }[/math]
- [math]\displaystyle{ \ F_{net//} = F_f - mgSinθ }[/math]
- [math]\displaystyle{ \ F_{netperp} = N -mgCosθ }[/math]
All energy equations are also present, especially when rotation is included on an inclined plane.
A Computational Model
Using GlowScript or Vpython we are able to model a box on an inclined plane moving upward with an initial velocity then pulled downward as it is being affected by gravity. This code allows us to observe the final velocity, position, speed, and acceleration of the box.
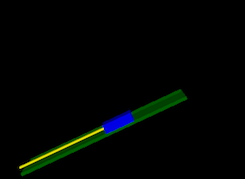
Code
from __future__ import division
from visual import *
from visual.graph import *
scene.title = "Incline Plane"
scene.background = color.black scene.center = (0.7, 1, 0) # location at which the camera looks
inclinedPlane = box(pos = vector(0.6, 0, 0), size = (1.2, 0.02, 0.2), color = color.green, opacity = 0.3)
cart = box(size = (0.2, 0.06, 0.06), color = color.blue) # 20-cm long cart on the inclined plane
trail = curve(color = color.yellow, radius = 0.01) # units are in meters
cart.m = 0.5 # mass of cart in kg
cart.pos = vector(0, 0.04, 0.08)
cart.v = vector(0, 0, 0) # initial velocity of car in (vx, vy, vz) form, units are m/s
theta = 25.0 * (pi / 180.0)
inclinedPlane.rotate(angle = theta, origin = (0, 0, 0), axis = (0,0,1)) cart.rotate(angle = theta, origin = (0, 0, 0), axis = (0,0,1))
cart.v = norm(inclinedPlane.axis) cart.v.mag = 3
g = 9.8 # acceleration due to gravity; units are m/s/s
mu = 0.20 # coefficient of friction between cart and plane
t = 0 #starting time deltat = 0.0005 # time step units are s
print "initial cart position (m): ", cart.pos
while cart.pos.y > 0.02 :
rate(1000) Fnet = norm(inclinedPlane.axis) Fnet.mag = -(cart.m * g * sin(theta)) if cart.v.y > 0: Fnet.mag += (mu * cart.m * g * cos(theta)) else: Fnet.mag -= (mu * cart.m * g * cos(theta)) cart.v = cart.v + (Fnet/cart.m * deltat)
cart.pos = cart.pos + cart.v * deltat
trail.append(pos = cart.pos) t = t + deltat
print "final time (s): ", t print "final cart position (m): ", cart.pos print "final cart velocity (m/s): ", cart.v print "final cart speed (m/s): ", mag(cart.v) print "final cart acceleration (m/s/s): ", (mag(Fnet) / cart.m)
Examples
The easiest example of an object on an inclined plane is removing all forces, but gravity. Since gravity is the only force acting on the object then the computations are easier. To make inclined plane problems harder, adding more forces or calculating for factors other than net force can be included such as finding the acceleration or time it takes for the block to go from the top to the bottom of an inclined plane. As well adding in rotation, with a ball rolling down the plane, would demonstrate other difficulties.
Easy
A 2.5 kg block slides down a plane inclined at an angle of 35 degrees to the horizontal. The coefficient of kinetic friction is 0.14.
a) draw a free body diagram for the block.
b) Determine the normal force exerted on the block.
- [math]\displaystyle{ \mathrm{N} = mgCosθ \, }[/math]
- [math]\displaystyle{ \mathrm{N} = 2.5*9.8*Cos35 \, }[/math]
- [math]\displaystyle{ \mathrm{N} = 20 N \, }[/math]
c) Determine the force of kinetic friction.
- [math]\displaystyle{ \mathrm{F_f} = mu*N \, }[/math]
- [math]\displaystyle{ \mathrm{F_f} = .14*20 \, }[/math]
- [math]\displaystyle{ \mathrm{F_f} = 2.8 N \, }[/math]
d) Determine the acceleration of the block.
- [math]\displaystyle{ \mathrm{F_net} = mgSinθ - F_ f = ma_x\, }[/math]
- [math]\displaystyle{ \mathrm{a_x} = \frac {mgSinθ - F_ f }{m}\, }[/math]
- [math]\displaystyle{ \mathrm{a_x} = \frac {24.5*Sin35 - 2.8}{2.5} \, }[/math]
- [math]\displaystyle{ \mathrm{a_x} = 4.5 m/s^2 \, }[/math]
Medium
Hard
Technical Usage
Terminology
Let's imagine there is a right triangle. The side opposite to the right angle is a Slant. The side on the bottom is Run. The side vertical to the bottom is Rise.
Slope: A slope brings a mechanical advantage to the incline plane.
- [math]\displaystyle{ \theta = \tan^{-1} \bigg( \frac {\text{Rise}}{\text{Run}} \bigg) \, }[/math]
Mechanical Advantage
Fw is a gravitational force that applies on the plane
Fi is a force exerted on the object and parallel to the plane
- [math]\displaystyle{ \mathrm{MA} = \frac{F_w}{F_i}. \, }[/math]
if the inclined plane is frictionless,
- [math]\displaystyle{ \text{MA} = \frac{F_w}{F_i} = \frac {1}{\sin \theta} \, }[/math] (in this case, [math]\displaystyle{ \sin \theta = \frac {\text{Rise}}{\text{Length}} \, }[/math])
if the inclined plane has a friction
- [math]\displaystyle{ \mathrm{MA} = \frac {F_w}{F_i} = \frac {\cos \phi} { \sin (\theta - \phi ) } \, }[/math] (in this case, [math]\displaystyle{ \phi = \tan^{-1} \mu \, }[/math])
- [math]\displaystyle{ \theta \lt \phi\, }[/math]: Downhill applied force is needed.
- [math]\displaystyle{ \theta = \phi\, }[/math]: Infinite mechanical advantage.
- [math]\displaystyle{ \theta \gt \phi\, }[/math]: The mechanical advantage is positive. Uphill force is needed.
History
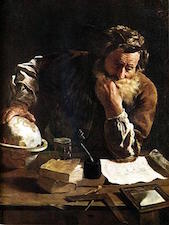
An inclined plane is one of the six classical simplified machines defined by Renaissance scientists. They have been used for thousands of years to move large objects to a specific distance, such as with the potential creation Stonehenge or the formation of the Egyptian Pyramids. In the second century B.C., Archimedes began theorizing some of the properties associated with an inclined plane including its various applications. Then in the first century A.D., Hero of Alexandria went beyond Archimedes initial theories to further explain the functionality of an inclined plane. These theories then proved to be a reality as the years went on and there were more advancements and functions applied to inclined planes.
Connectedness
Every day people experience some sort of inclined plane. From driving up a hill on the way to work to walking down a ramp to get to class (because of all of the construction we always have) an inclined plane is a vital piece of our lives. What I find the most interesting about inclined planes is how people use them and physics for fun without even knowing it. Such as going down a slide at a park or skiing down a snowy mountain, the physics and forces behind an inclined plane bring enjoyment to many people's lives every day.
An inclined plane relates to mechanical engineering because MEs will always be working with various systems including some form of an inclined plane. Such as pulling an object across a ramp or the mechanics behind a water slide at an amusement park, inclined planes are everywhere. The best advice is to become comfortable with how they work and how to calculate the net force acting on the object sitting or moving on the inclined plane.
See Also
Free Body Diagram
Normal Force
References
Cole, Matthew (2008). Explore science, 2nd Ed. Pearson Education. https://books.google.com/books?id=RhuciGEQ1G8C&pg=PA178#v=onepage&q&f=false
http://hyperphysics.phy-astr.gsu.edu/hbase/Mechanics/incline.html